This article will allow you to develop and implement your own shopping cart utilizing a Session, a DataGrid, and the
DataTable
class of theDataSet
object. Through this article, you'll learn to:- Build a user interface using ASP.NET Web Server Controls
- Dynamically construct a DataTable depending on user interaction
- Bind the dynamically constructed DataTable to a DataGrid
- Allow the user to remove items from the cart freely
- Keep a running cost total of items within the cart
At the end of this article, you'll have a fully functioning shopping cart, and you'll have gained a thorough understanding of DataTables, DataGrids, and Session Variables. Download a complete demonstration of the final product here.
This project is separated into five parts:
- Building the user interface
- Building the DataTable structure
- Adding items to the cart
- Keeping a running total
- Removing items from the cart
Step 1: Building the Shopping Cart User Interface
The user interface or UI for the application is quite simple. In fact, most of the interaction will occur within the DataGrid, but the UI does include some key components that the user will be interacting with:
- A
DropDownList
Web control that will display the products that we'll offer. The cost of each item will be associated with the value of theDropDownList
Web control for simplicity's sake. - A
TextBox
Web control that offers the user the ability to adjust quantities - A
Button
Web control to add to the cart - A
DataGrid
that will contain the cart's contents - A
Label
control that will display to the user a running total in terms of price
Now that you have an idea of what the UI will display, let's add these components to the body of an HTML page. Using Dreamweaver MX, we'll create a new page and add this code into the
<body>
tag of the page:<form runat="server">
Product:<br>
<asp:DropDownList id="ddlProducts" runat="server">
<asp:ListItem Value="4.99">Socks</asp:ListItem>
<asp:ListItem Value="34.99">Pants</asp:ListItem>
<asp:ListItem Value="14.99">Shirt</asp:ListItem>
<asp:ListItem Value="12.99">Hat</asp:ListItem>
</asp:DropDownList><br>
Quantity:<br>
<asp:textbox id="txtQuantity" runat="server" /><br><br>
<asp:Button id="btnAdd" runat="server" Text="Add To Cart"
onClick="AddToCart" /><br><br>
<asp:DataGrid id="dg" runat="server" /><br><br>
Total:
<asp:Label id="lblTotal" runat="server" />
</form>
The code is actually quite simple and needs very little explanation. Basically, a hard-coded
DropDownList
control (ddlProducts
)
is added, with four products. The cost of those products is maintained by associating a decimal value for each specific product.Second, a
TextBox
control (txtQuantity
) is added so that the user can modify quantities. Next, a Button
control (btnAdd
) is added with the text "Add to Cart". The onClick
event associated with the Button control will call the AddToCart()
subroutine, which will be created in the next section.Next, a
DataGrid
(dg
) is added which will be used to bind to the dynamically constructed DataTable
. Remember, the DataTable
will be constructed in code, and bound to the DataGrid
for presentation to the user. We'll add a button column to allow the user to remove a specific item if he or she wishes a little later. Finally, we'll add a Label
control (lblTotal
), which will be used to display to the user a running total of the items within the cart.Step 2: Building the DataTable
Structure
If you're familiar with DataSets
,
then you know that DataTables provide you with a way to dynamically create a purely memory-resident representation of a database table. Typically, you'd fill a DataTable
from an existing database, but you could also create one programmatically, as will be the case here.In a DataTable, columns are represented by the columns property, and rows are represented by the rows property. Thus, DataTables will be the perfect choice for the creation of our shopping cart. We can build the columns just as we would within a database, using the columns property of the
DataTable, and add rows to the DataTable with the Rows
property. With the DataTable built, we can then bind the DataTable to a DataGrid to display the results in an intuitive manner.Because DataTables contain rows and columns, you will be able to effectively mock the structure of a conventional database table. The rows will be added to the DataTable as the user adds items to the cart. For now, we'll need to construct the columns that will serve as the categories for the row items. In order for the cart to function correctly, we'll need to add the following columns with a corresponding data type:
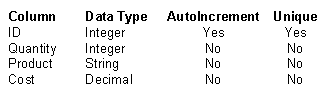
You're probably wondering how data types, auto increment, and uniqueness will be set programmatically. Remember, DataTables contain column and row properties. Some of those properties include the ability to set the above mentioned items, just as you would a traditional database table. You'll also notice that the
DataTable
contains a column for ID. Technically, this column has nothing to do with the shopping cart, but it will have a lot to do with keeping the items in the cart unique, and will allow us to establish a primary key if we ever want to create a relationship with another DataTable.For now we just want the structure of the DataTable built when the page loads for the first time. We don't want to actually start to define rows until the user selects an item to add to the cart.
To begin building the cart's structure, add this code into the head of your page:
<script runat="server">
Dim objDT As System.Data.DataTable
Dim objDR As System.Data.DataRow
Private Sub Page_Load(s As Object, e As EventArgs)
If Not IsPostBack Then
makeCart()
End If
End Sub
Function makeCart()
objDT = New System.Data.DataTable("Cart")
objDT.Columns.Add("ID", GetType(Integer))
objDT.Columns("ID").AutoIncrement = True
objDT.Columns("ID").AutoIncrementSeed = 1
objDT.Columns.Add("Quantity", GetType(Integer))
objDT.Columns.Add("Product", GetType(String))
objDT.Columns.Add("Cost", GetType(Decimal))
Session("Cart") = objDT
End Function
</script>
Looking at the code, you can see, that the
makeCart()
function is called only when the page is loaded for the first time. This is the reason for the IsPostBack check.Within the
makeCart()
function, we'll add the code that defines the actual structure for the DataTable and its columns. First, we add a column to the DataTable named ID
, assigning it the data type for integer. We assign the property for AutoIncrement
to True
, and begin the seed at 1.Next, add three more columns to the DataTable for
Quantity
,Product
, and Cost
, assigning them the data types for integer, string, and decimal respectively. Finally, the DataTable is added into aSession
conveniently named "Cart
", for storage.That's it! If you think about the structure of a database table and then consider the structure and code for the DataTable, they begin to resemble each other conceptually. The next step involves adding items to the cart, which is no harder than defining new rows for the DataTable.
Thank you vikash this very useful.....
ReplyDelete